Compilers
Question 1 |
Generation of intermediate code based on an abstract machine model is useful in compilers because
it makes implementation of lexical analysis and syntax analysis easier | |
syntax-directed translations can be written for intermediate code generation | |
it enhances the portability of the front end of the compiler | |
it is not possible to generate code for real machines directly from high level language programs |
Question 2 |
Type checking is normally done during
lexical analysis | |
syntax analysis | |
syntax directed translation | |
code optimization |
Question 3 |
The number of tokens in the following C statement.
printf("i = %d, &i = %x", i, &i);
is
3 | |
26 | |
10 | |
21 |
(i) Keyword
(ii) Identifier
(iii) Constant
(iv) Variable
(v) String
(vi) Operator
Print = Token 1
( = Token 2
"i=%d%x" = Token 3 [Anything inside " " is one Token]
, = Token 4
i = Token 5
, = Token 6
& = Token 7
i = Token 8
) = Token 9
; = Token 10
Here, totally 10 Tokens are present in the equation.
Question 4 |
For the program segment given below, which of the following are true?
program main (output); type link = ^data; data = record d : real; n : link end; var ptr : link; begin new (ptr); ptr:=nil; .ptr^.d:=5.2; write ln(ptr) end.
The program leads to compile time error | |
The program leads to run time error | |
The program outputs 5.2 | |
The program produces error relating to nil pointer dereferencing | |
None of the above |
Question 5 |
In a compiler the module the checks every character of the source text is called:
The code generator. | |
The code optimizer. | |
The lexical analyser. | |
The syntax analyser. |
Question 6 |
Using longer identifiers in a program will necessarily lead to:
Somewhat slower compilation | |
A program that is easier to understand | |
An incorrect program | |
None of the above |
Question 7 |
Consider a program P that consists of two source modules M1 and M2 contained in two different files. If M1 contains a reference to a function defined in M2, the reference will be resolved at
Edit time | |
Compile time | |
Link time | |
Load time |
Question 8 |
Match the following:
(P) Lexical analysis (1) Graph coloring (Q) Parsing (2) DFA minimization (R) Register allocation (3) Post-order traversal (S) Expression evaluation (4) Production tree
P-2, Q-3, R-1, S-4 | |
P-2, Q-1, R-4, S-3 | |
P-2, Q-4, R-1, S-3 | |
P-2, Q-3, R-4, S-1 |
Q) Expression can be evaluated with postfix traversals.
R) Register allocation can be done by graph colouring.
S) The parser constructs a production tree.
Hence, answer is ( C ).
Question 9 |
Match the following:
(P) Lexical analysis (i) Leftmost derivation (Q) Top down parsing (ii) Type checking (R) Semantic analysis (iii) Regular expressions (S) Runtime environments (iv) Activation records
P ↔ i, Q ↔ ii, R ↔ iv, S ↔ iii | |
P ↔ iii, Q ↔ i, R ↔ ii, S ↔ iv | |
P ↔ ii, Q ↔ iii, R ↔ i, S ↔ iv | |
P ↔ iv, Q ↔ i, R ↔ ii, S ↔ iii |
Top down parsing has left most derivation of any string.
Type checking is done in semantic analysis.
Activation records are loaded into memory at runtime.
Question 10 |
Match the following according to input (from the left column) to the compiler phase (in the right column) that processes it:
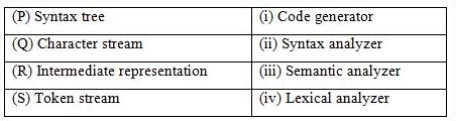
P→(ii), Q→(iii), R→(iv), S→(i) | |
P→(ii), Q→(i), R→(iii), S→(iv) | |
P→(iii), Q→(iv), R→(i), S→(ii) | |
P→(i), Q→(iv), R→(ii), S→(iii) |
Token stream is forwarded as input to Syntax analyzer which produces syntax tree as output. So, S → (ii).
Syntax tree is the input for the semantic analyzer, So P → (iii).
Intermediate representation is input for Code generator. So R → (i).
Question 11 |
Which one of the following statements is FALSE?
Context-free grammar can be used to specify both lexical and syntax rules. | |
Type checking is done before parsing. | |
High-level language programs can be translated to different Intermediate Representations. | |
Arguments to a function can be passed using the program stack. |