Compiler-Design
Question 1 |

Which one of the following choices represents the correct combination for the numbered cells in the parsing table (“blank” denotes that the corresponding cell is empty)?
A | ① S ⟶ Rf ② S ⟶ Rf ③ T ⟶ ∊ ④ T ⟶ ∊
|
B | ① blank ② S ⟶ Rf ③ T ⟶ ∊ ④ T ⟶ ∊ |
C | ① S ⟶ Rf ② blank ③ blank ④ T ⟶ ∊ |
D | ① blank ② S ⟶ Rf ③ blank ④ blank |
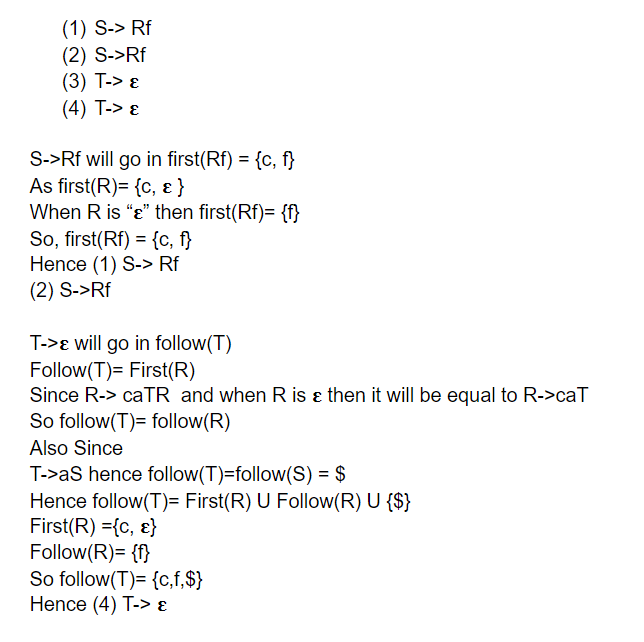
Question 2 |
P ⟶ D* E*
D ⟶ int ID {record that ID.lexeme is of type int}
D ⟶ bool ID {record that ID.lexeme is of type bool}
E ⟶ E1 + E2 {check that E1.type = E2.type = int; set E.type := int}
E ⟶ !E1 {check that E1.type = bool; set E.type := bool}
E ⟶ ID {set E.type := int}
With respect to the above grammar, which one of the following choices is correct?
A | The actions can be used to type-check syntactically correct integer variable declarations and integer expressions. |
B | The actions will lead to an infinite loop. |
C | The actions can be used to type-check syntactically correct boolean variable declarations and boolean expressions. |
D | The actions can be used to correctly type-check any syntactically correct program. |
Question 3 |
S1:Every SLR(1) grammar is unambiguous but there are certain unambiguous grammars that are not SLR(1).
S2: For any context-free grammar, there is a parser that takes at most O(n3 )
Which one of the following options is correct?
A | S1is true and S2is true
|
B | S1is true and S2is false |
C | S1is false and S2is true |
D | S1is false and S2is false |
Every unambiguous grammar need not be SLR(1). As we know some unambiguous grammar which is CLR(1) but not SLR(1). So S1 is true.
Any CFG (which is in CNF form) can be parsed by CYK algorithm in O(n3) where n is length of string. Although it is not given that CFG is in CNF form but since we can convert any CFG in CNF form so S2 is true
Question 4 |
a = b + c;
e = a + 1;
d = b + c;
f = d + 1;
g = e + f;
In a compiler, this code segment is represented internally as a directed acyclic graph (DAG). The number of nodes in the DAG is _______
A | 6 |
Question 5 |
For the program segment given below, which of the following are true?
program main (output); type link = ^data; data = record d : real; n : link end; var ptr : link; begin new (ptr); ptr:=nil; .ptr^.d:=5.2; write ln(ptr) end.
A | The program leads to compile time error |
B | The program leads to run time error |
C | The program outputs 5.2 |
D | The program produces error relating to nil pointer dereferencing |
E | None of the above |
Question 6 |
A part of the system software, which under all circumstances must reside in the main memory, is:
A | text editor |
B | assembler |
C | linker |
D | loader |
E | none of the above |
Question 7 |
Suppose we have a computer with a single register and only three instructions given below:
LOAD addren ; load register ; from addren STORE addren ; store register ; at addren ADD addren ; add register to ; contents of addren ; and place the result ; in the register
Consider the following grammar:
A → id :=E E → E + T|T T → (E)|id
Write a syntax directed translation to generate code using this grammar for the computer described above.
A | Theory Explanation. |
Question 8 |
Match the following items

A | (i) - (d), (ii) - (a), (iii) - (b), (iv) - (c) |
Yacc (Yet Another Compiler- Compiler) is a computer program for the UNIX operating system. It is a LALR parser generator, generating a parser, the part of a compiler that tries to make syntactic sense of the source code, specially a LALR parser, based on an analytic grammar. Yacc is written in portable C.
Question 9 |
Generation of intermediate code based on an abstract machine model is useful in compilers because
A | it makes implementation of lexical analysis and syntax analysis easier |
B | syntax-directed translations can be written for intermediate code generation |
C | it enhances the portability of the front end of the compiler |
D | it is not possible to generate code for real machines directly from high level language programs |
Question 10 |
Construct the LL(1) table for the following grammar.
1. Expr → _Expr 2. Expr → (Expr) 3. Expr → Var Expr Tail 4. ExprTail → _Expr 5. ExprTail → λ 6. Var → Id Var Tail 7. VarTail → (Expr) 8. VarTail → λ 9. Goal → Expr$
A | Theory Explanation. |
Question 11 |
(a) Translate the arithmetic expression a*-(b+c) into syntax tree.
(b) A grammar is said to have cycles if it is the case that
A ⇒ +A
Show that no grammar that has cycles can be LL(I).
A | Theory Explanation. |
Question 12 |
A shift reduce parser carries out the actions specified within braces immediately after reducing with the corresponding rule of grammar
S → xxW {print "1"} S → y {print "2"} W → Sz {print "3"}
What is the translation of xxxxyzz using the syntax directed translation scheme described by the above rules?
A | 23131 |
B | 11233 |
C | 11231 |
D | 33211 |

⇒ 23131
Note SR is bottom up parser.
Question 13 |
A linker is given object modules for a set of programs that were compiled separately. What information need to be included in an object module?
A | Object code |
B | Relocation bits |
C | Names and locations of all external symbols defined in the object module
|
D | Absolute addresses of internal symbols |
To link to external symbols it must know the location of external symbols.
Question 14 |
Consider the productions A⟶PQ and A⟶XY. Each of the five non-terminals A, P, Q, X, and Y has two attributes: s is a synthesized attribute, and i is an inherited attribute. Consider the following rules.
Rule 1: P.i = A.i + 2, Q.i = P.i + A.i, and A.s = P.s + Q.s Rule 2: X.i = A.i + Y.s and Y.i = X.s + A.i
Which one of the following is TRUE?
A | Only Rule 2 is L-attributed.
|
B | Neither Rule 1 nor Rule 2 is L-attributed. |
C | Both Rule 1 and Rule 2 are L-attributed. |
D | Only Rule 1 is L-attributed.
|
Question 15 |
Consider the following statements.
- I. Symbol table is accessed only during lexical analysis and syntax analysis.
II. Compilers for programming languages that support recursion necessarily need heap storage for memory allocation in the run-time environment.
III. Errors violating the condition ‘any variable must be declared before its use’ are detected during syntax analysis.
Which of the above statements is/are TRUE?
A | II only |
B | I only |
C | I and III only
|
D | None of I, II and III |
II is wrong as compilers which supports recursion require stack memory in run time environment.
III is wrong “any variable must be declared before its use” is a semantic error and not syntax error.
Question 16 |
Consider the following grammar.
S → aSB|d B → b
The number of reduction steps taken by a bottom-up parser while accepting the string aaadbbb is _______.
A | 7 |

7 reductions total.
Question 17 |
Consider the syntax-directed translation schema (SDTS) shown below:
E → E + E {print “+”} E → E ∗ E {print “.”} E → id {print id.name} E → (E)
An LR-parser executes the actions associated with the productions immediately after a reduction by the corresponding production. Draw the parse tree and write the translation for the sentence.
(a+b)∗(c+d), using the SDTS given above.
A | Theory Explanation. |
Question 18 |
Given below are the transition diagrams for two finite state machine M1 and M2 recognizing languages L1 and L2 respectively.
(a) Display the transition diagram for a machine that recognizes L1.L2, obtained from transition diagrams for M1 and M2 by adding only ε transitions and no new states.
(b) Modify the transition diagram obtained in part(a) obtain a transition diagram for a machine that recognizes (L1.L2)∗ by adding only ε transitions and no new states. (Final states are enclosed in double circles).

A | Theory Explanation. |
Question 19 |
Which of the following macros can put a micro assembler into an infinite loop?
(i) .MACRO M1 X .IF EQ, X ;if X=0 then M1 X + 1 .ENDC .IF NE X ;IF X≠0 then .WORD X ;address (X) is stored here .ENDC .ENDM (ii) .MACRO M2 X .IF EQ X M2 X .ENDC .IF NE, X .WORD X+1 .ENDC .ENDM
A | (ii) only |
B | (i) only |
C | both (i) and (ii) |
D | None of the above |
Question 20 |
The pass number for each of the following activities
- 1. Object code generation
2. Literals added to literal table
3. Listing printed
4. Address resolution of local symbols
That occur in a two pass assembler respectively are
A | 1, 2, 1, 2 |
B | 2, 1, 2, 1 |
C | 2, 1, 1, 2 |
D | 1, 2, 2, 2 |
Pass 1:
1) Assign addresses to all statements in the program.
2) Save the values assigned to all labels for use in pass 2.
3) Perform some processing of assembler directives.
Pass 2:
1) Assemble instructions.
2) Generate data values defined by BYTE, WORD etc.
3) Perform processing of assembler directives not done during pass 1.
4) Write the program and assembling listing.
Question 21 |
Consider the following grammar and the semantic actions to support the inheritance type declaration attributes. Let X1, X2, X3, X4, X5 and X6 be the placeholders for the non-terminals D, T, L or L1 in the following table:

Which one of the following are the appropriate choices for X1, X2, X3 and X4?
A | X1 = L, X2 = L, X3 = L1, X4 = T |
B | X1 = L, X2 = T, X3 = L1, X4 = L |
C | X1 = T, X2 = L, X3 = L1, X4 = T |
D | X1 = T, X2 = L, X3 = T, X4 = L1 |
L → L1, id {X3.type = X4.type } , this production has L and L1, hence X3 and X4 cannot be T.
So option 1, 3 and 4 cannot be correct.
Hence, 2 is correct answer.
Question 22 |
Consider the augmented grammar given below:
S' → S S → 〈L〉 | id L → L,S | S
Let I0 = CLOSURE ({[S' → ·S]}). The number of items in the set GOTO (I0 , 〈 ) is: _____.
A | 4 |
B | 5 |
C | 6 |
D | 7 |

Hence, the set GOTO (I0 , 〈 ) has 5 items.
Question 23 |
Which one of the following kinds of derivation is used by LR parsers?
A | Leftmost in reverse |
B | Rightmost in reverse |
C | Leftmost |
D | Rightmost |
Question 24 |
S → Aa
A → BD
B → b | ε
D → d | ε
Let a, b, d and $ be indexed as follows:
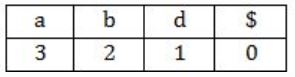
Compute the FOLLOW set of the non-terminal B and write the index values for the symbols in the FOLLOW set in the descending order. (For example, if the FOLLOW set is {a, b, d, $}, then the answer should be 3210)
A | 30 |
B | 31 |
C | 10 |
D | 21 |
{Follow(B) = Follow(A) when D is epsilon}
Follow(B) = {d} Union {a} = {a,d}
Hence Answer is : 31
Question 25 |
A language L allows declaration of arrays whose sizes are not known during compilation. It is required to make efficient use of memory. Which of the following is true?
A | A compiler using static memory allocation can be written for L |
B | A compiler cannot be written for L; an interpreter must be used |
C | A compiler using dynamic memory allocation can be written for L |
D | None of the above |
Question 26 |
The conditional expansion facility of macro processor is provided to
A | test a condition during the execution of the expanded program |
B | to expand certain model statements depending upon the value of a condition during the execution of the expanded program |
C | to implement recursion |
D | to expand certain model statements depending upon the value of a condition during the process of macro expansion |
Question 27 |
Heap allocation is required for languages
A | that support recursion |
B | that support dynamic data structures |
C | that use dynamic scope rules |
D | None of the above |
Question 28 |
In the following grammar
X ::= X ⊕ Y/Y Y ::= Z * Y/Z Z ::= id
Which of the following is true?
A | ‘⊕’ is left associative while ‘*’ is right associative |
B | Both ‘⊕’ and ‘*’ is left associative |
C | ‘⊕’ is right associative while ‘*’ is left associative |
D | None of the above |

⊕ is left associative.
* is right associative.
Question 29 |
Let the attribute 'val' give the value of a binary number generated by S in the following grammar:
S → L.L | L L→ LB | B B → 0 | 1
For example, an input 101.101 gives S.val = 5.625
Construct a syntax directed translation scheme using only synthesized attributes, to determine S.val.
A | Theory Explanation. |
Question 30 |
In a resident – OS computer, which of the following systems must reside in the main memory under all situations?
A | Assembler |
B | Linker |
C | Loader |
D | Compiler |
Some OS may allow virtual memory may allow the loader to be located in a region of memory that is in page table.
Question 31 |
Which of the following statements is true?
A | SLR parser is more powerful than LALR |
B | LALR parser is more powerful than Canonical LR parser |
C | Canonical LR parser is more powerful than LALR parser |
D | The parsers SLR, Canonical CR, and LALR have the same power |
Canonical LR parser is more powerful than LALR parser.
Question 32 |
Type checking is normally done during
A | lexical analysis |
B | syntax analysis |
C | syntax directed translation |
D | code optimization |
Question 33 |
Let synthesized attribute val give the value of the binary number generated by S in the following grammar. For example, on output 101.101, S.val = 5.625.
S → LL|L L → LB|B B → 0|1
Write S-attributed values corresponding to each of the productions to find S.val.
A | Theory Explanation. |
Question 34 |
The number of tokens in the Fortran statement DO 10 I = 1.25 is
A | 3 |
B | 4 |
C | 5 |
D | None of the above |
10 → 2
I → 3
= → 4
1.25 → 5
Question 35 |
Which of the following is the most powerful parsing method?
A | LL (1) |
B | Canonical LR |
C | SLR |
D | LALR |
LR > LALR > SLR
Question 36 |
Consider the syntax directed translation scheme (SDTS) given in the following.
Assume attribute evaluation with bottom-up parsing, i.e., attributes are evaluated immediately after a reduction.
E → E1 * T {E.val = E1.val * T.val} E → T {E.val = T.val} T → F – T1{T.val = F.val – T1.val} T → F {T.val = F.val} F → 2 {F.val = 2} F → 4 {F.val = 4}
(a) Using this SDTS, construct a parse tree for the expression
4 – 2 – 4 * 2
and also compute its E.val.
(b) It is required to compute the total number of reductions performed to parse a given input. Using synthesized attributes only, modify the SDTS given, without changing the grammar, to find E.red, the number of reductions performed while reducing an input to E.
A | Theory Explanation is given below. |
Question 37 |
Given the following expression grammar:
E → E * F | F + E | F F → F - F | id
which of the following is true?
A | * has higher precedence than + |
B | - has higher precedence than * |
C | + and – have same precedence |
D | + has higher precedence than * |
Order of precedence is *, +, -.
Here * and + have equal preference, '-' can have higher precedence than + and *.
Question 38 |
The number of tokens in the following C statement.
printf("i = %d, &i = %x", i, &i);
is
A | 3 |
B | 26 |
C | 10 |
D | 21 |
(i) Keyword
(ii) Identifier
(iii) Constant
(iv) Variable
(v) String
(vi) Operator
Print = Token 1
( = Token 2
"i=%d%x" = Token 3 [Anything inside " " is one Token]
, = Token 4
i = Token 5
, = Token 6
& = Token 7
i = Token 8
) = Token 9
; = Token 10
Here, totally 10 Tokens are present in the equation.
Question 39 |
Which of the following derivations does a top-down parser use while parsing an input string? The input is assumed to be scanned in left to right order.
A | Leftmost derivation |
B | Leftmost derivation traced out in reverse |
C | Rightmost derivation |
D | Rightmost derivation traced out in reverse |
Bottom-Up parser - Reverse of rightmost derivation
Question 40 |
(a) Remove left-recursion from the following grammar:
S → Sa| Sb | a | b
(b) Consider the following grammar:
S → aSbS| bSaS |ε
Construct all possible parse trees for the string abab. Is the grammar ambiguous?
A | Theory Explanation is given below. |
Question 41 |
Consider the following grammar with terminal alphabet ∑{a,(,),+,*} and start symbol E. The production rules of the grammar are:
E → aA E → (E) A → +E A → *E A → ε
(a) Compute the FIRST and FOLLOW sets for E and A.
(b) Complete the LL(1) parse table for the grammar.
A | Theory Explanation is given below. |
Question 42 |
The syntax of the repeat-until statement is given by the gollowing grammar
S → repeat S1 until E
Where E stands for expressions, S and S1 stand for statement. The non-terminals S and S1 have an attribute code that represents generated code. The nonterminal E has two attributes. The attribute code represents generated code to evaluate the expression and store its truth value in a distinct variable, and the attribute varName contains the name of the variable in which the truth value is stored? The truth-value stored in the variable is 1 if E is true, 0 if E is false.
Give a syntax-directed definition to generate three-address code for the repeatuntil statement. Assume that you can call a function newlabel( ) that returns a distinct label for a statement. Use the operator ‘\\’ to concatenate two strings and the function gen(s) to generate a line containing the string s.
A | Theory Explanation is given below. |
Question 43 |
The process of assigning load addresses to the various parts of the program and adjusting the code and date in the program to reflect the assigned addresses is called
A | Assembly |
B | Parsing |
C | Relocation |
D | Symbol resolution |
Question 44 |
Which of the following statements is false?
A | An unambiguous grammar has same leftmost and rightmost derivation |
B | An LL(1) parser is a top-down parser |
C | LALR is more powerful than SLR |
D | An ambiguous grammar can never be LR(k) for any k |
Option C: LALR is more powerful than SLR.
Option D: An ambiguous grammar can never be LR (k) for any k, because LR(k) algorithm aren’t designed to handle ambiguous grammars. It would get stuck into undecidability problem, if employed upon an ambiguous grammar, no matter how large the constant k is.
Question 45 |
(a) Construct all the parse trees corresponding to i + j * k for the grammar
E → E+E E → E*E E → id
- (b) In this grammar, what is the precedence of the two operators * and +?
(c) If only one parse tree is desired for any string in the same language, what changes are to be made so that the resulting LALR(1) grammar is non-ambiguous?
A | Theory Explanation is given below. |
Question 46 |
1: a?(b∣c)*aT
2: b?(a∣c)*bT
3: c?(b∣a)*c
Note that ‘x?’ means 0 or 1 occurrence of the symbol x. Note also that the analyzer outputs the token that matches the longest possible prefix.
If the string bbaacabc is processes by the analyzer, which one of the following is the sequence of tokens it outputs?
A | T1T2T3 |
B | T1T1T3 |
C | T2T1T3 |
D | T3T3 |
T1 : (b+c)*a + a(b+c)*a
T2 : (a+c)*b + b(a+c)*b
T3 : (b+a)*c + c(b+a)*c
Now the string is: bbaacabc
Please NOTE:
Token that matches the longest possible prefix
We can observe that the longest possible prefix in string is : bbaac which can be generated by T3.
After prefix we left with “abc” which is again generated by T3 (as longest possible prefix).
So, answer is T3T3.
Question 47 |
Consider the following parse tree for the expression a#b$c$d#e#f, involving two binary operators $ and #.
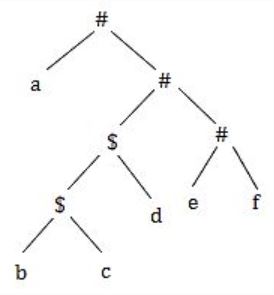
Which one of the following is correct for the given parse tree?
A | $ has higher precedence and is left associative; # is right associative |
B | # has higher precedence and is left associative; $ is right associative
|
C | $ has higher precedence and is left associative; # is left associative |
D | # has higher precedence and is right associative; $ is left associative
|
Question 48 |
Which one of the following statements is FALSE?
A | Context-free grammar can be used to specify both lexical and syntax rules. |
B | Type checking is done before parsing. |
C | High-level language programs can be translated to different Intermediate Representations. |
D | Arguments to a function can be passed using the program stack. |
Question 49 |
Consider the following grammar for arithmetic expression using binary operators – and/which are not associative:
E → E−T|T T → T/F|F F → (E)id(E is the start symbol)
(a) Is this grammar unambiguous? If so, what is the relative precedence between – and/if not, give an unambiguous grammar that gives/precedence over -
(b) Does the grammar allow expressions with redundant parentheses as in (id/id) or in id-(id/id)? If so, convert the grammar into one which does not generate expressions with redundant parentheses. Do this with minimum number of changes to the given production rules and adding at most one more production rule.
A | Theory Explanation. |
Question 50 |
Choose the correct alternatives (more than one may be correct) and write the corresponding letters only: A “link editor” is a program that:
A | matches the parameters of the macro-definition with locations of the parameters of the macro call |
B | matches external names of one program with their location in other programs |
C | matches the parameters of subroutine definition with the location of parameters of subroutine call |
D | acts as link between text editor and the user |
E | acts as a link between compiler and user program |
1) external symbol resolution
2) relocation