Control Flow
Question 1 |
What is the following program segment doing?
main()
{
int d=1;
do
{
printf(“%d”\n”,d++);
}while(d<=9);
}
main()
{
int d=1;
do
{
printf(“%d”\n”,d++);
}while(d<=9);
}
Adding 9 integers | |
Adding integers from 1 to 9 | |
Displaying integers from 1 to 9 | |
No output |
Question 1 Explanation:
The code consists of do-while loop in which action performs first and later condition checking.
In the printf() statement, d++ means first it will display d value and increment the d value later condition checking.So the integer values 1 to 9 will be printed.
In the printf() statement, d++ means first it will display d value and increment the d value later condition checking.So the integer values 1 to 9 will be printed.
Question 2 |
How many lines of output does the following C code produce?
#include<stdio.h>
float i=2.0;
float j=1.0;
float sum = 0.0;
main()
{
while (i/j > 0.001)
{
j+=j;
sum=sum+(i/j);
printf("%f\n", sum);
}
}
#include<stdio.h>
float i=2.0;
float j=1.0;
float sum = 0.0;
main()
{
while (i/j > 0.001)
{
j+=j;
sum=sum+(i/j);
printf("%f\n", sum);
}
}
8 | |
9 | |
10 | |
11 |
Question 2 Explanation:
Iteration-1:
while (1.000000 > 0.001)
{
j=2.0
sum=0+1.000000;
printf("%f\n",sum); /* It will print 1.000000 */
}
Iteration-2: 1.500000
Iteration-3: 1.750000
Iteration-4: 1.875000
Iteration-5: 1.937500
Iteration-6: 1.968750
Iteration-7: 1.984375
Iteration-8: 1.992188
Iteration-9: 1.996094
Iteration-10: 1.998047
Iteration-11: 1.999023
The program will terminate after 11th iteration. So, it print 11 lines.
while (1.000000 > 0.001)
{
j=2.0
sum=0+1.000000;
printf("%f\n",sum); /* It will print 1.000000 */
}
Iteration-2: 1.500000
Iteration-3: 1.750000
Iteration-4: 1.875000
Iteration-5: 1.937500
Iteration-6: 1.968750
Iteration-7: 1.984375
Iteration-8: 1.992188
Iteration-9: 1.996094
Iteration-10: 1.998047
Iteration-11: 1.999023
The program will terminate after 11th iteration. So, it print 11 lines.
Question 3 |
Consider the following segment of C-code:
The number of comparisons made in the execution of the loop for any n > 0 is:

The number of comparisons made in the execution of the loop for any n > 0 is:
⌊log 2n⌋*n | |
n | |
⌊log 2n⌋ | |
⌊log 2n⌋+1 |
Question 3 Explanation:
Explanation:
Let us consider n=6, then
1<=6 (correct)
2<=6 (correct)
4<=6 (correct)
8<=6 (False)
4 comparisons required
Option A:
⌊log n⌋+1
⌊log 6⌋+1
3+1=4 (correct)
Option B:
n=6 (False)
Option C:
⌊log n⌋
⌊log 6⌋=3 (False)
Option D:
⌊log 2n⌋+1
⌊log 26⌋+1=2+1=3 (False)
Let us consider n=6, then
1<=6 (correct)
2<=6 (correct)
4<=6 (correct)
8<=6 (False)
4 comparisons required
Option A:
⌊log n⌋+1
⌊log 6⌋+1
3+1=4 (correct)
Option B:
n=6 (False)
Option C:
⌊log n⌋
⌊log 6⌋=3 (False)
Option D:
⌊log 2n⌋+1
⌊log 26⌋+1=2+1=3 (False)
Question 4 |
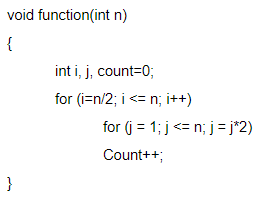
The complexity of the program is
O(log n) | |
O(n2) | |
O(n2 log n) | |
O(n log n) |
Question 4 Explanation:
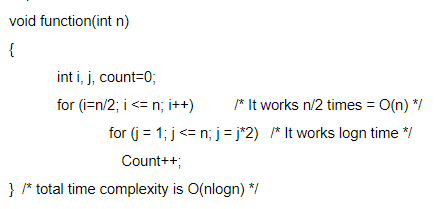
Question 5 |
What does the following program do when the input is unsigned 16-bit integer?
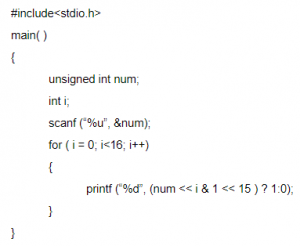
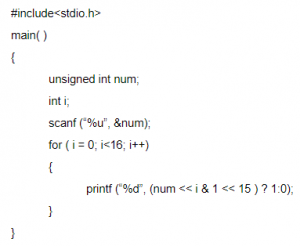
It prints all even bits from num | |
It prints all odd bits from num | |
It prints binary equivalent of num | |
None of the above |
Question 5 Explanation:
Step-1: Take n value initially 14(any number we can take but we are taken 14)
Step-2: 14<16
Step-3: It will print 00000000 00001110
Step-2: 14<16
Step-3: It will print 00000000 00001110