C-Programming
Question 1 |
What is printed by the following ANSI C program?
#include
int main(int argc, char *argv[])
{
int x = 1, z[2] = {10, 11};
int *p = NULL;
p = &x;
*p = 10;
p = &z[1];
*(&z[0] + 1) += 3;
printf("%d, %d, %d\n", x, z[0], z[1]);
return 0;
}
#include
int main(int argc, char *argv[])
{
int x = 1, z[2] = {10, 11};
int *p = NULL;
p = &x;
*p = 10;
p = &z[1];
*(&z[0] + 1) += 3;
printf("%d, %d, %d\n", x, z[0], z[1]);
return 0;
}
A | 1, 10, 11 |
B | 1, 10, 14 |
C | 10, 14, 11 |
D | 10, 10, 14 |
Question 1 Explanation:
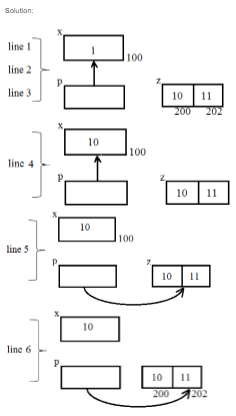
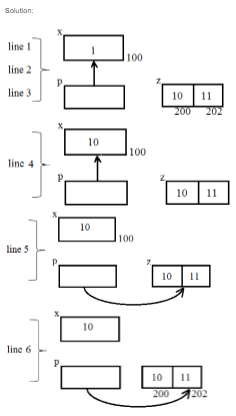
Question 2 |
Which of the following is wrong about the data types?
A | The number is always positive when qualifier 'unsigned' is used. |
B | The number can be positive or negative when the qualifier 'signed' is used. |
C | The range of values for signed data types is more than that of unsigned data types |
D | The left most bit in unsigned data type is used to represent the value |
Question 2 Explanation:
Except statement-3 all statements are true
Question 3 |
Given i= 0, j = 1, k = –1, x = 0.5, y = 0.0 What is the output of given ‘C’ expression ?
x * 3 && 3 || j | k
x * 3 && 3 || j | k
A | -1 |
B | 0 |
C | 1 |
D | 2 |
Question 3 Explanation:
Step-1: Evaluate x * 3 because multiplication has more priority than remaining operators x * 3→ 1.5
Step-2: && is logical AND. Both the statements are TRUE, then it returns 1 otherwise 0.
1.5 && 3 is TRUE. So, it returns 1.
Step-3: j | k is bitwise OR operator. It returns -1.
Step-4: ((x * 3) && 3) || (j | k) islogical OR operator. It returns 1
Note: The precedence is ((x * 3) && 3) || (j | k)
Step-2: && is logical AND. Both the statements are TRUE, then it returns 1 otherwise 0.
1.5 && 3 is TRUE. So, it returns 1.
Step-3: j | k is bitwise OR operator. It returns -1.
Step-4: ((x * 3) && 3) || (j | k) islogical OR operator. It returns 1
Note: The precedence is ((x * 3) && 3) || (j | k)
Question 4 |
___ is a parameter passing method that waits to evaluate the parameter value until it is used?
A | Pass by value |
B | Pass by name |
C | Pass by reference |
D | Pass by pointer |
Question 4 Explanation:
Pass by value: The method parameter values are copied to another variable and then the copied object is passed, that’s why it’s called pass by value.
Pass by reference: An alias or reference to the actual parameter is passed to the method.Passing a pointer to the actual parameter, and indirect through the pointer
Pass by name: re-evaluate the actual parameter on every use. For actual parameters that are simple variables, this is the same as call by reference. For actual parameters that are expressions, the expression is re-evaluated on each access.
There is no parameter passing method with the name “ Pass by pointer”
Pass by reference: An alias or reference to the actual parameter is passed to the method.Passing a pointer to the actual parameter, and indirect through the pointer
Pass by name: re-evaluate the actual parameter on every use. For actual parameters that are simple variables, this is the same as call by reference. For actual parameters that are expressions, the expression is re-evaluated on each access.
There is no parameter passing method with the name “ Pass by pointer”
Question 5 |
The quantity of very long word is:
A | 32 bits |
B | 64 bits |
C | 128 bits |
D | 256 bits |
Question 5 Explanation:
A double word is a single unit of data expressing two adjacent words (a word is a standard unit of data for a certain processor architecture). For instance, if a single word is 16-bits in size, a double word would be 32-bits. A double word can doubled a second time, which turns it into a very long word that is 64-bits.
Question 6 |
The library function exit() causes an exit from the loop in which it occurs
A | The loop in which it occurs |
B | The block in which it occurs |
C | The function in which it occurs |
D | none of these |
Question 6 Explanation:
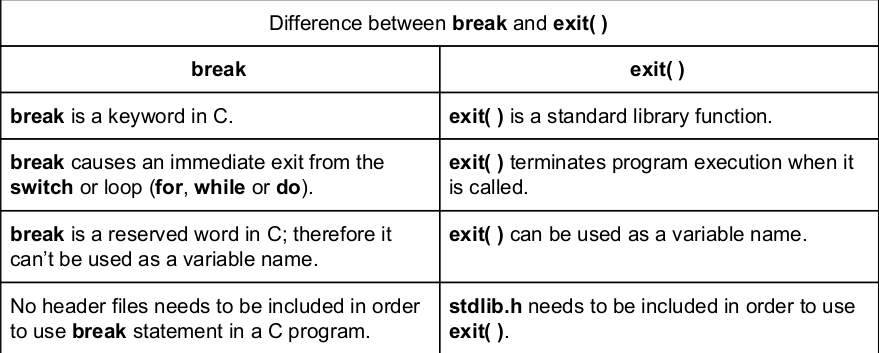

Question 7 |
The IOS class member function used for formatting IO is:
A | Width(), precision(), read() |
B | Width(), precision(), setf() |
C | getch(),width(),Io() |
D | unsetf(),setf(),write() |
Question 7 Explanation:
Table : describes the functions of ios class in brief.
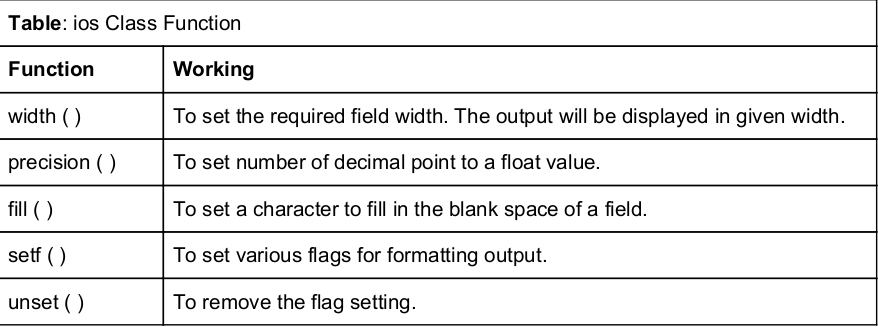
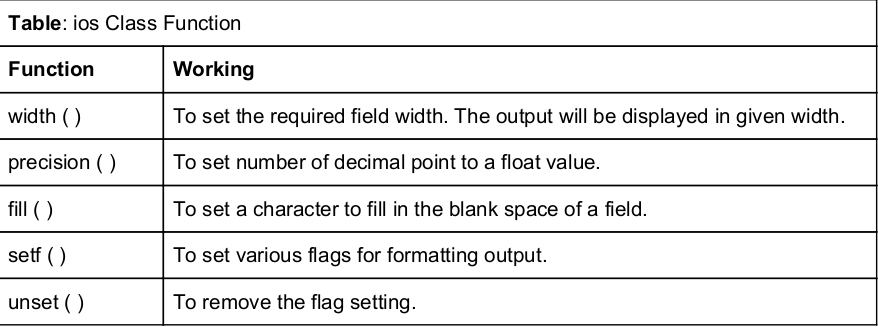
Question 8 |
Consider the following C-code fragment running on a 32-bit x86 machine:
typedef struct
{
union
{
Unsigned char a;
unsigned short b;
}
unsigned char c;
}S;
S B[10];
S*p=&B[4];
S*q=&B[5];
p→ U.b=0x1234;
/* structure S takes 32-bits */
If M is the value of q-p and N is the value of ((int) & (p→ c))-((int)p), then (M,N) is
typedef struct
{
union
{
Unsigned char a;
unsigned short b;
}
unsigned char c;
}S;
S B[10];
S*p=&B[4];
S*q=&B[5];
p→ U.b=0x1234;
/* structure S takes 32-bits */
If M is the value of q-p and N is the value of ((int) & (p→ c))-((int)p), then (M,N) is
A | (1,1)
|
B | (3,2)
|
C | (1,2) |
D | (4,4) |
Question 8 Explanation:
#include
typedef struct{
union
{
unsigned char a;
unsigned short b;
}U;
unsigned char c;
}S;
int main()
{
printf("Hello World");
S B[10]; // declaring array of 10 structures
S*p=&B[4]; // Assigning 4th array value to pointer p
S*q=&B[5]; // Assigning 5th array value to pointer q
p-> U.b=0x1234; // Assigning a value to 4th structure array element's union short b
printf("q-p %u\n",sizeof(p)); // value is 1, as pointer subtraction formula is (address of q - address of p) / size of structure
printf("%d\n",((int) & (p->c))-((int)p)); // value is 2 the address difference between p starting address and ‘c’ address, between them is only short integer of 2 bytes
return 0;
}
typedef struct{
union
{
unsigned char a;
unsigned short b;
}U;
unsigned char c;
}S;
int main()
{
printf("Hello World");
S B[10]; // declaring array of 10 structures
S*p=&B[4]; // Assigning 4th array value to pointer p
S*q=&B[5]; // Assigning 5th array value to pointer q
p-> U.b=0x1234; // Assigning a value to 4th structure array element's union short b
printf("q-p %u\n",sizeof(p)); // value is 1, as pointer subtraction formula is (address of q - address of p) / size of structure
printf("%d\n",((int) & (p->c))-((int)p)); // value is 2 the address difference between p starting address and ‘c’ address, between them is only short integer of 2 bytes
return 0;
}
Question 9 |
The ASCII code 56, represents the character
A | V |
B | 8 |
C | a |
D | carriage return |
Question 10 |
If a variable can take only integral values from 0 to n, where n is a constant integer, then the variable can be represented as a bit-field whose width is the integral part of (the log in the answers are to the base 2)
A | log (n) + 1 |
B | log (n-1) + 1 |
C | log (n+1) + 1 |
D | none of the above |
Access subject wise (1000+) question and answers by becoming as a solutions adda PRO SUBSCRIBER with Ad-Free content
Register Now
You have completed
questions
question
Your score is
Correct
Wrong
Partial-Credit
You have not finished your quiz. If you leave this page, your progress will be lost.
Correct Answer
You Selected
Not Attempted
Final Score on Quiz
Attempted Questions Correct
Attempted Questions Wrong
Questions Not Attempted
Total Questions on Quiz
Question Details
Results
Date
Score
Hint
Time allowed
minutes
seconds
Time used
Answer Choice(s) Selected
Question Text
Need more practice!
Keep trying!
Not bad!
Good work!
Perfect!