Arrays
Question 1 |
Consider the following C program.
#includeint main () { int a [4] [5] = {{1, 2, 3, 4, 5}, {6, 7, 8, 9, 10}, {11, 12, 13, 14, 15}, {16, 17, 18, 19, 20}}; printf (“%d\n”, *(*(a+**a+2) +3)); return (0); }
The output of the program is _______.
19 |
#include
int main()
{
int a[4][5] = { {1,2,3,4,5},
{6,7,8,9,10},
{11,12,13,14,15},
{16,17,18,19,20}
};
printf("%d\n",a); //880 (consider base address = 880)
printf("%d\n",*a); //880
printf("%d\n",**a); //1
printf("%d\n",**a+2); //3
printf("%d\n",a+**a+2); //940
printf("%d\n",*(a+**a+2));//940
printf("%d\n",*(a+**a+2)+3);//952
printf("%d\n",*(*(a+**a+2)+3));//19
return 0;
}

Question 2 |
Let A be a two dimensional array declared as follows:
A: array [1 ... 10] [1 ... 15] of integer;
Assuming that each integer takes one memory location, the array is stored in row-major order and the first element of the array is stored at location 100, what is the address of the element a[i][j]?
15i + j + 84 | |
15j + i + 84 | |
10i + j + 89 | |
10j + i + 89 |
100 + 15 * (i-1) + (j-1)
= 100 + 15i - 15 + j - 1
= 15i + j + 84
Question 3 |
Suppose you are given an array s[1...n] and a procedure reverse (s,i,j) which reverses the order of elements in a between positions i and j (both inclusive). What does the following sequence do, where 1 ≤ k ≤ n:
reverse(s, 1, k) ; reverse(s, k + 1, n); reverse(s, l, n);
Rotates s left by k positions | |
Leaves s unchanged | |
Reverses all elements of s | |
None of the above |
Question 4 |
Consider the following declaration of a two dimensional array in C:
char a[100][100];
Assuming that the main memory is byte-addressable and that the array is stored starting from memory address 0, the address of a [40][50] is:
4040 | |
4050 | |
5040 | |
5050 |
= 0 + [40 * 100 * 1] + [50 * 1]
= 4000 + 50
= 4050
Question 5 |
Assume the following C variable declaration
int *A [10], B[10][10];
Of the following expressions
I. A[2] II. A[2][3] III. B[1] IV. B[2][3]
which will not give compile-time errors if used as left hand sides of assignment statements in a C program?
I, II, and IV only | |
II, III, and IV only | |
II and IV only | |
IV only |
ii) A[2][3] This results an integer, no error will come.
iii) B[1] is a base address of an array. This will not be changed it will result a compile time error.
iv) B[2][3] This also results an integer. No error will come.
Question 6 |
#include
{
int a[3][3][3] =
{{1, 2, 3, 4, 5, 6, 7, 8, 9},
{10, 11, 12, 13, 14, 15, 16, 17, 18},
{19, 20, 21, 22, 23, 24, 25, 26, 27}};
int i = 0, j = 0, k = 0;
for( i = 0; i < 3; i++ ){
for(k = 0; k < 3; k++ )
printf("%d ", a[i][j][k]);
printf("\n");
}
return 0;
}
1 2 3 10 11 12 19 20 21 | |
1 4 7 10 13 16 19 22 25 | |
1 2 3 4 5 6 7 8 9 | |
1 2 3 13 14 15 25 26 27 |
Hence, 1, 2, 3 will be 1^st row
10 , 11, 12 will be 2^nd row
19, 20, 21 will be 3^rd row
Question 7 |
A Young tableau is a 2D array of integers increasing from left to right and from top to bottom. Any unfilled entries are marked with ∞, and hence there cannot be any entry to the right of, or below a ∞. The following Young tableau consists of unique entries.
1 2 5 14 3 4 6 23 10 12 18 25 31 ∞ ∞ ∞
When an element is removed from a Young tableau, other elements should be moved into its place so that the resulting table is still a Young tableau (unfilled entries may be filled in with a ∞). The minimum number of entries (other than 1) to be shifted, to remove 1 from the given Young tableau is ____________.
4 | |
5 | |
6 | |
7 |

Question 8 |
Consider the following C program:
#include <stdio.h> int main () { int arr [] = {1,2,3,4,5,6,7,8,9,0,1,2,5}, *ip = arr+4; printf ("%d\n", ip[1]); return 0; }
The number that will be displayed on execution of the program is _____.
5 | |
6 | |
7 | |
8 |
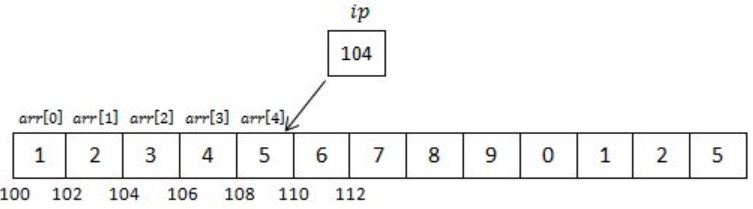
We know that arr is a pointer to arr[ ] & hence arr+4 is pointer to 4th index of array (starting from 0 to 4).
Now *ip is a pointer of int type pointing to memory location 108, which is part of arr.
Hence, when we will print ip[1] it will be equivalent to *(ip+1).
Address of ip will be incremented by 1 & value inside 110 will be printed.
Question 9 |
A program P reads in 500 integers in the range [0, 100] representing the scores of 500 students. It then prints the frequency of each score above 50. What would be the best way for P to store the frequencies?
An array of 50 numbers | |
An array of 100 numbers | |
An array of 500 numbers | |
A dynamically allocated array of 550 numbers |
→ Then using array of 50 numbers is the best way to store the frequencies.
Question 10 |
Consider the list of numbers 1, 2, 3, ..., 1000 is stored in a[0..999]. What will be the total number of comparisons to search x = 501 using the following binary_search() function?
int binary_search(int a[ ], int n, int x)
{
int low=0, high=n-1;
while(low <= high)
{
int m = (low + high) /2:
if(x > a[m])
low = m+1;
else if(x < a[m])
high= m-1:
else
return m;
}
return -1;
}
2 | |
15 | |
17 | |
1 |
Question 11 |
P[2][5] | |
*(*(P+2)+5) | |
*(P[2]+5)
| |
*(P+2+5) |
P[2][5] represents the element present in column five of row two. So it can be used to access the required given element in question.
Option (B):
*(*(P+2)+5)
*(P+2) → will lead to enter row two.
(*(P+2)+5) will give address of element present at fifth column of row 2.
→ *(*(P+2)+5) will return element present at fifth column of row 2.
Hence option (B) is Correct.
Option (C): *(P[2]+5)
P[2] → will give starting address of row 2
P[2]+5 → will give address of element present at 5th column of row 2.
*(P[2]+5) → will return the element present at 5th column of row 2.
Hence it is correct option.
Option (D): *(P+2+7)
= (P+7)
*(P+7) will return starting address of 7th row.
→ Hence it is not the correct answer.
Question 12 |
Which of the following statements is INCORRECT with respect to pointers declared in the following ‘C’ language code?
Void main()
Int a[10], *p, *q;
p=&a[5];
q=&a[7];
}
Q-p | |
P+1 | |
Q-3 | |
P+q |
Question 13 |
2048
| |
0 | |
1024 | |
2049 |
→Example:[-2:2] consists of -2,-1,0,1,2 indexes.
Question 14 |
15 | |
16 | |
3 | |
17 |
Question 15 |
{ int a|5| = {2,3}; Printf (“/n%d%d%d”, a[2], a[3], a[4]) ; }
Garbage values | |
2 3 3
| |
3 2 2 | |
0 0 0 |